"Do a barrel roll"
Circuit
This example shows how to prepare custom JQuery script to control element without use of framework UI elements. Connect potentiometers to pin A0 and A1.
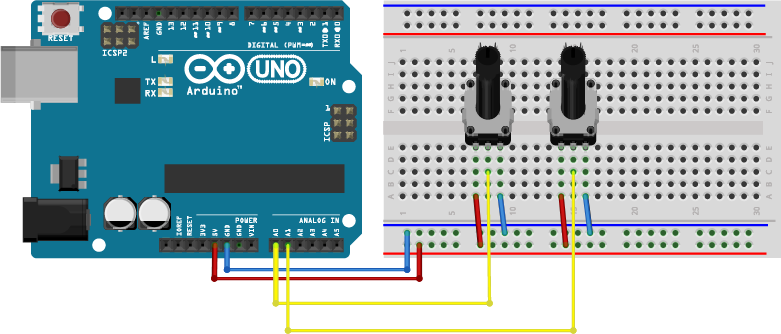
Edit the Involt Arduino sketch
void loop(){
involtReceive();
involtSend(0, analogRead(A0));
delay(2);
involtSend(1, analogRead(A1));
delay(2);
}...
HTML & CSS
In this tutorial we need big square at the center of app screen. First add HTML code in app.html to create square.
Create custom JavaScript file and CSS.
<link rel="stylesheet" type="text/css" href="customstyle.css">
Add the CSS:
margin: 200px auto;
width: 200px;
height: 200px;
background: gray;
}JQuery
Interval based script
The final step is real-time manipulation of square. Use set interval to automatically update data. Each analog pin value is stored in involt.pin.A[index] array where index is the pin number. The 50ms delay is used to lower the CPU usage.
$(".square").css({
"width": involt.pin.A[0],
"height": involt.pin.A[0],
"transform": "rotate("+involt.pin.A[1]+"deg)"
});
},50);Now open your App and test the script. You should be able to rotate the square.
Listen for pin
You should be able to do the same thing with listening for pin function. The involtListenForPin must be defined for each pin separately.
Important: because it will update the square each time the value of pins are received, you must add higher delay in your arduino sketch.
$(".square").css({
"width": value,
"height": value
});
};involtListenForPin[1] = function(index, value){
$(".square").css({
"transform": "rotate("+value+"deg)"
});
};